Integrating Firebase with Node.js: A Comprehensive Guide
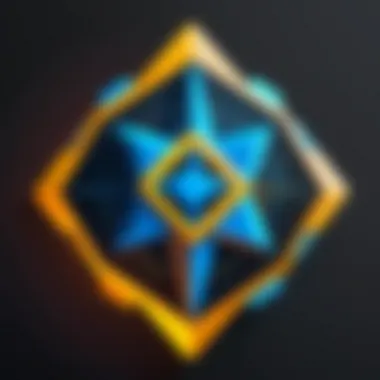
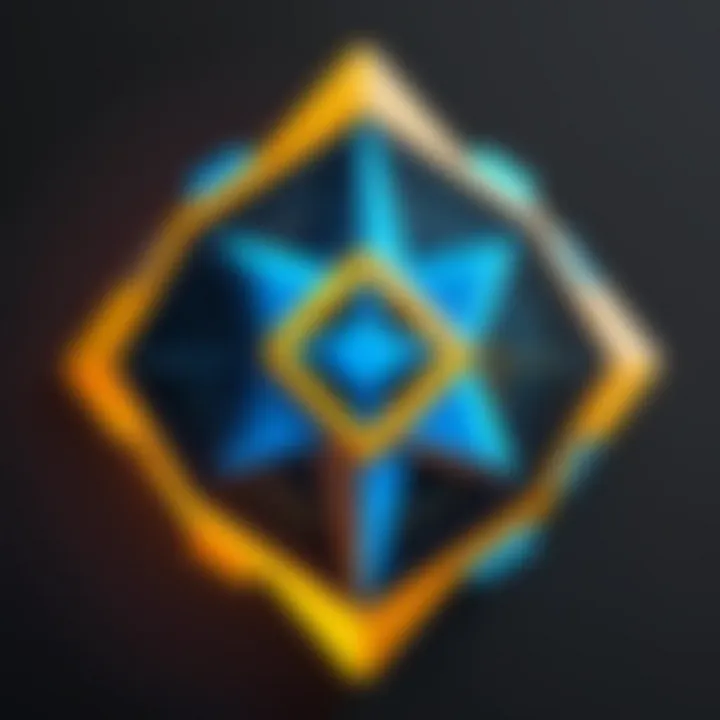
Intro
Firebase has emerged as a powerful platform for building web and mobile applications. This is particularly true when paired with Node.js, a popular server-side runtime. Together, they form a robust combination that enables developers to create applications with dynamic functionalities. In this guide, we will explore how to effectively integrate Firebase with Node.js, providing step-by-step instructions and best practices.
To start, we will discuss the principal features of Firebase and how they enhance a Node.js-based application. Understanding these features can help developers leverage Firebaseโs capabilities, making their applications more efficient and user-focused.
Next, we will dive into setting up a Firebase project, ensuring that developers have the necessary foundation before implementing core functionalities such as authentication and real-time database interactions. Itโs imperative to grasp these components, as they provide versatility and dynamism to the overall user experience.
As we advance, we will delve into more complex topics such as cloud functions and optimizing performance. These elements are critical for scaling applications that require robust back-end support. Ultimately, this guide aims to furnish readers with knowledge and practical skills necessary to master the integration of Firebase with Node.js.
Prolusion to Firebase
Firebase plays a critical role in modern application development, offering a suite of services aimed at simplifying the backend and improving efficiency. It provides tools that facilitate real-time data synchronization, user authentication, and cloud functions which are vital for building scalable applications. For developers integrating Firebase with Node.js, understanding Firebase's core functionalities lays the groundwork for effective application design.
What is Firebase?
Firebase is a platform developed by Google to help developers build applications more efficiently. Originally an independent company, Firebase offers a host of backend services accessible through simple APIs. These include the Realtime Database, Cloud Firestore, Authentication, Hosting, and more. The platform enables developers to leverage various services without having to manage server infrastructure, which streamlines the development process considerably. This allows developers to focus on creating engaging user experiences rather than getting bogged down in server management.
Key Features of Firebase
Firebase comes with numerous features that distinguish it from traditional backends:
- Realtime Database: Offers real-time data synchronization across all connected clients, making it ideal for applications that require live updates.
- Cloud Firestore: A flexible, scalable database that allows for complex queries and is optimized for mobile and web applications.
- Firebase Authentication: Simplifies user authentication processes with multiple methods including email/password, Google, Facebook, and more.
- Cloud Functions: Run backend code in response to events triggered by Firebase features and HTTPS requests, allowing for a serverless architecture.
- Hosting: Provides fast and secure static hosting for web applications, ensuring a smooth deployment process.
These features collectively enhance the capabilities of an application, leading to quicker development cycles and a more dynamic user experience.
Benefits of Using Firebase
Integrating Firebase into a Node.js application offers several benefits:
- Rapid Development: The ready-to-use services offered by Firebase significantly reduce the time required to set up backend infrastructure.
- Scalability: Firebase is built to scale effortlessly, handling an increase in users and data volume without much adjustment.
- Real-time Capabilities: The ability to push updates to users instantly increases the interactivity and engagement of applications.
- Cross-Platform Compatibility: Firebase supports multiple platforms allowing developers to build web, iOS, and Android applications simultaneously with a unified backend.
- Robust Security Features: With built-in security rules and authentication options, Firebase helps maintain user data integrity and privacy.
In summary, Firebase serves as a powerful ally for developers looking to integrate backend services in a Node.js environment. Its features not only provide essential functionalities but also enhance overall development experiences, making it easier to innovate and deliver high-quality applications.
Intro to Node.js
The integration of Firebase with Node.js represents a potent combination in modern web development. Knowing about Node.js is essential for developers to effectively utilize Firebaseโs capabilities. Node.js provides a server-side environment based on JavaScript, enabling asynchronous communication and performance efficiency. This section will elaborate on Node.js, offering insights into its foundation and relevance within the Firebase context.
What is Node.js?
Node.js is an open-source JavaScript runtime built on Chrome's V8 engine. It allows developers to use JavaScript on the server side. This unifies the programming language across the stack, making development more streamlined. Unlike traditional server-side solutions, it operates on a non-blocking, event-driven architecture. This means it can handle multiple requests simultaneously, making it particularly suitable for real-time applications like those powered by Firebase.
Key Concepts of Node.js
Node.js introduces several key concepts that differentiates it from other back-end technologies:
- Event-Driven Architecture: Instead of waiting for tasks to complete, Node.js utilizes an event loop to manage operations. This results in higher throughput and lower latency.
- Non-Blocking I/O: Operations for file and network requests do not block other operations from executing. This enhances performance, particularly under heavy load.
- Single-Threaded Model: While it runs on a single thread, Node.js can leverage multiple cores through clustering, allowing it to scale effectively.
Understanding these concepts is crucial for any developer venturing into integrating Firebase with Node.js.
Advantages of Node.js
Node.js offers numerous advantages which make it a compelling choice for developers, especially when combined with Firebase:
- High Performance: The non-blocking nature translates to exceptional performance for I/O-bound applications.
- Unified Language: Using JavaScript across both front-end and back-end simplifies the learning process, reducing context switching for developers.
- Rich Ecosystem: Node.js benefits from a large community and a wealth of libraries and frameworks available via npm (Node Package Manager).
- Rapid Development: The ability to build applications quickly allows developers to focus on core functionality rather than boilerplate code.
"Node.js is not just a tool, but a platform that empowers developers to create scalable applications rapidly."
In summary, the choice of Node.js in conjunction with Firebase not only enhances the developer experience but also optimizes application performance. This introduction ties directly into the next section, where we delve deeper into setting up a Firebase project.
Setting Up Firebase with Node.js
Setting up Firebase with Node.js is a foundational step for building modern web applications that require real-time features and robust data management. Firebase provides efficient back-end services, enabling easier development and deployment of applications. By integrating Firebase with Node.js, developers can leverage the benefits of both platforms, streamlining processes such as authentication, database management, and cloud functions. This integration is crucial as it helps in handling asynchronous operations smoothly while maintaining performance.
Creating a Firebase Project
Creating a Firebase project is the first step to harness the power of Firebase within a Node.js application. Head over to Firebase Console and sign in with your Google account. Once logged in, click on "Add Project" to begin.
During the setup, you will be prompted to name your project and choose whether to enable Google Analytics. Although itโs an option, it can be skipped initially to focus on the essential setup. Following the project creation, you will have access to the project dashboard where critical settings and tools are available.
After this, configure your web app. This will generate Firebase configuration details such as , , and others, which are key for initiating Firebase services within your Node.js application. Store these parameters securely to ensure they are easily accessible when needed.
Installing Firebase SDK
Once the project is created, the next step is installing the Firebase SDK. This is essential because the SDK includes all the necessary tools to interact with Firebase services. To install the Firebase SDK, open a terminal in the root directory of your Node.js project and run the following command:
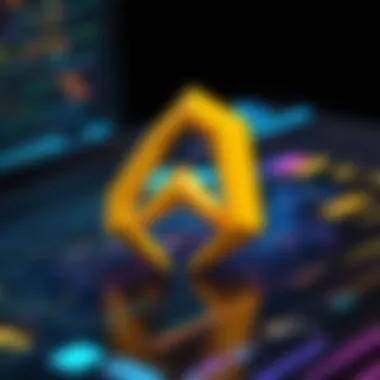
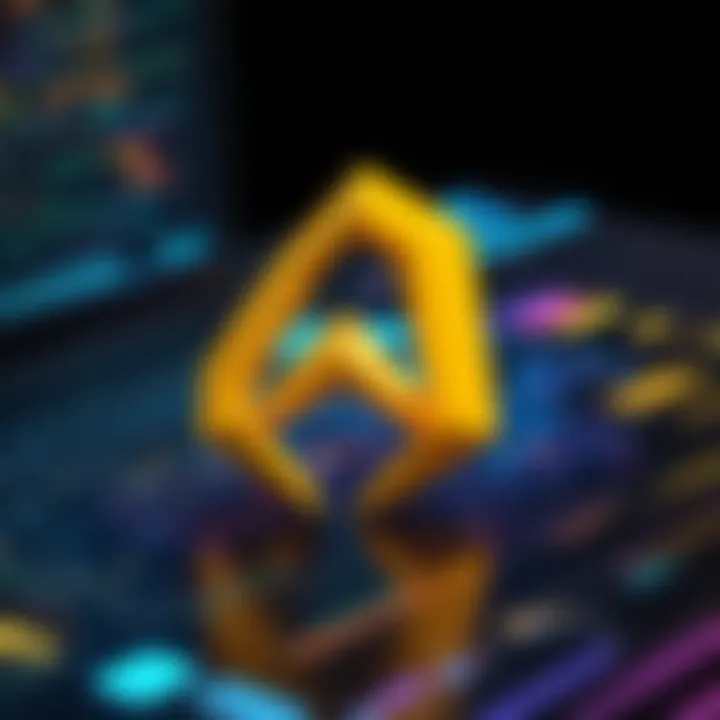
This command fetches the latest version of the Firebase package and adds it to your project dependencies. The SDK allows you to integrate various features such as Realtime Database, Authentication, and more. Ensure that you keep your SDK updated to benefit from the latest features and security updates.
Connecting Node.js to Firebase
With the Firebase SDK installed, you can now connect your Node.js application to Firebase. Begin by importing the Firebase module in your application file (usually or ). The code below exemplifies how to initialize Firebase with the configuration parameters you collected during project setup:
Ensure that all provided configuration variables correspond with those from your Firebase console. Once the connection is established, your Node.js application can begin to interact with Firebase services efficiently. This setup lays the groundwork for further functionalities, such as real-time databases and authentication mechanisms.
Connecting Node.js to Firebase is a key step to enabling your application to take full advantage of the features provided by Firebase.
Implementing Firebase Realtime Database
The Firebase Realtime Database is a crucial component in the Firebase ecosystem, allowing developers to build applications that require real-time data synchronization and data storage. This capability is especially relevant in today's landscape where user demands for instantaneous updates are high. Real-time database functionality not only enhances user experience but also provides a seamless interaction model across different devices. Understanding how to implement this feature is vital for developers aiming to create scalable and efficient systems.
When integrating Firebase Realtime Database with Node.js, several key aspects need attention. First, the ease of writing and retrieving data is a cornerstone of this tool. Developers must ensure data integrity and security while leveraging the platformโs capabilities. This integration empowers applications to reflect changes instantaneously, thus meeting user expectations for responsiveness. Here, we will elaborate on the specific components involved in setting up and effectively using Firebase Realtime Database.
Setting Up Database Rules
Before any interaction with the Firebase Realtime Database, setting up database rules is essential. These rules determine who can access the database and what data they can read or write. By defining rules, you can enforce security policies that protect sensitive information and ensure proper data handling.
Database rules are expressed in JSON format, making it intuitive for developers familiar with both JavaScript and the data structure of Firebase. Hereโs a simple example of a read/write rule:
In the rule above, only authenticated users can read or write to the database. Adjusting these rules is necessary as the application evolves. Continuous monitoring and updating security rules can prevent unauthorized access, ensuring that user data remains secure.
Writing Data to the Database
Once the database rules are established, the next step is writing data to the Firebase Realtime Database. Data is written to the database in JSON structure. Using Node.js, a typical write operation could look like this:
This code snippet illustrates how to set data in a specific path within the database. It is essential to structure data correctly, especially when working with nested data and complex structures. Developers should be mindful of how data organization affects retrieval performance.
Reading Data from the Database
Reading data from the Firebase Realtime Database is straightforward yet powerful. By using the Firebase API, developers can query data based on key parameters. For example:
This operation retrieves data from a specific location in the database and logs it to the console. Such operations can also listen for real-time updates. This feature significantly enhances interactivity and maintains application state consistency across user sessions.
Real-time Data Updates
Utilizing real-time capabilities allows applications to respond to data changes without requiring manual refreshes. This is particularly beneficial in scenarios like messaging apps or collaborative tools. Firebase handles this through event listeners. An example of listening for changes looks like this:
Using the method ensures that any updates to the specified data path will trigger the listener, thus maintaining updated local data. This feature ultimately provides a more engaging user experience, aligning with modern user expectations.
Firebase Authentication
Firebase Authentication plays a crucial role in the ecosystem of Firebase, providing a robust framework for managing user identities and access. With the increasing need for secure applications, understanding Firebase Authentication is essential for developers. It simplifies the complex processes involved in user management while offering an array of authentication methods out-of-the-box. The key benefits include enhanced security, user convenience, and ease of integration. Furthermore, it allows developers to focus more on building features rather than worrying about the intricacies of user authentication.
Methods of Authentication
Firebase supports various methods of authentication, allowing flexibility in how users can log in to applications. Understanding these methods is vital for implementing secure and user-friendly experiences. The primary methods include:
- Email/Password Authentication: This traditional approach remains popular due to its simplicity. Users can easily create accounts with their email and a password.
- Phone Authentication: Users can authenticate using their phone numbers, receiving an SMS verification code. This method is useful for users without access to email.
- Social Media Providers: Firebase enables integration with various third-party social media platforms like Facebook, Google, and Twitter. This streamlines user registration and login, attracting more users.
- Anonymous Authentication: Useful for applications where user identification is not immediately necessary. Users can access features without creating an account initially.
Implementing Email/Password Authentication
Implementing Email/Password authentication in Firebase is straightforward and requires minimal setup. Developers start by enabling this method in the Firebase console. After configuration, they can use Firebase SDK to manage the authentication process. Here is a brief step-by-step:
- Enable Email/Password Sign-in: Go to the Authentication section of the Firebase console. Under Sign-in Method, toggle on the email/password option.
- Create User Account: Developers can create a user account programmatically using:
- Sign in Users: Users can sign in with the correct email and password using:
This straightforward approach allows developers to implement a core functionality needed in any application while maintaining security.
Using Third-party Providers
Firebase Authentication integration with third-party providers offers a major convenience for users. Popular services include Facebook, Google, Twitter, and GitHub. Using these providers leverages existing user credentials which enhances user experience significantly. The integration process generally follows this pattern:
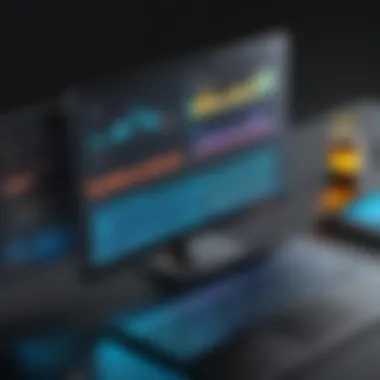
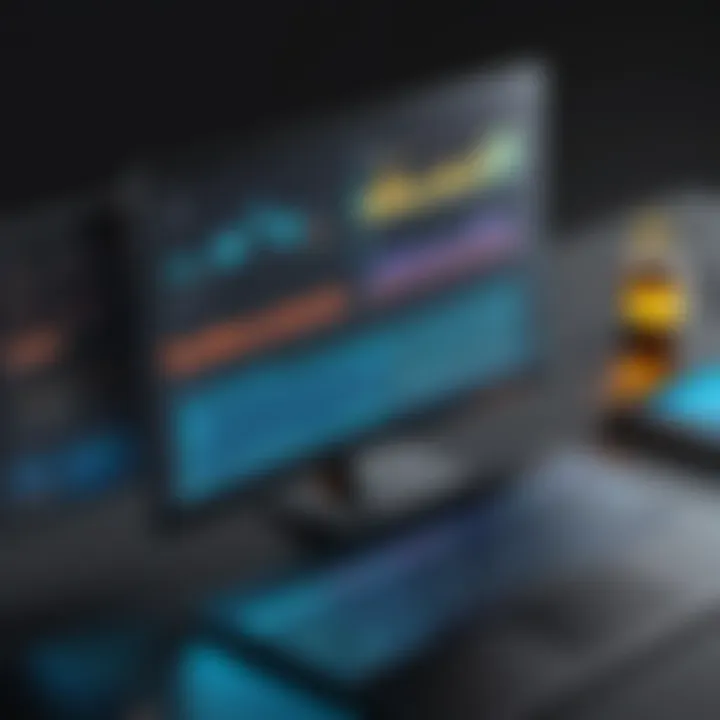
- Enable the provider in Firebase Console: Select your desired provider and configure it accordingly.
- Set Up the SDK: Use Firebase SDK to handle authentication requests. For example, integrating Google sign-in involves something like this:
- Handle User Information: Post-authentication, developers can easily access user details provided by the third-party service.
Using third-party authentication methods not only reduces friction during sign-up but also encourages users to engage with your application.
"Embracing Firebase Authentication empowers developers to build applications that prioritize user security and convenience, ultimately leading to greater user satisfaction."
By understanding and implementing Firebase Authentication, developers can create secure, user-friendly applications that harness the full potential of Firebase's offerings.
Utilizing Cloud Functions
Utilizing Cloud Functions stands as a pivotal topic within this guide. These functions allow developers to run backend code in response to events triggered by Firebase features or HTTPS requests. With Cloud Functions, configuring server infrastructure is not necessary. This leads to a more streamlined workflow, as developers can focus more on code than server management.
What are Cloud Functions?
Cloud Functions is a serverless execution environment provided by Firebase. It enables developers to write JavaScript functions that automatically run in the cloud. These functions can respond to events, such as database changes or user authentication. The beauty of Cloud Functions lies in its scalability; it automatically scales with the application's needs, allowing developers to handle varying loads of requests seamlessly.
Creating and Deploying Functions
Creating Cloud Functions involves a few structured steps:
- Set up Firebase CLI: If the Firebase CLI is not installed, download and install it.
- Initialize Functions in Project: Inside your Firebase project directory, runThis command will prepare your project for adding functions.
- Write Your Function: Navigate to the directory. Functions are typically written in . Here is a sample function that triggers on HTTP requests:
- Deploy Functions: Finally, deploy your function using:This puts your function live and accessible.
Triggering Functions with Events
Cloud Functions can be triggered by various Firebase services. Common triggers include:
- Realtime Database: Executes code when data is added, modified, or deleted.
- Firestore: Similar to Realtime Database but designed for more complex querying needs.
- Authentication: Activates functions when users sign up or log in.
- Analytics and In-app Messaging: Allows event-driven functions that can enhance user engagement.
Understanding these relationships makes it easier to implement and utilize Cloud Functions effectively. By harnessing these functions, developers can build more dynamic, interactive applications while maintaining a focus on core functionalities over infrastructure management.
Data Management Best Practices
Data management is a foundational aspect of software development, particularly when working with services like Firebase in conjunction with Node.js. Effective data management practices not only enhance application performance but also improve data integrity and security. It allows developers to create a structured design that can easily accommodate changes and scale as necessary.
Employing best practices in data management ensures that applications are resilient and efficient. This topic brings together various strategies aimed at maintaining a logical structure within your Firebase databases while enabling smooth interaction between your database and Node.js. It entails proper structuring of databases, efficient querying, and the capability to handle concurrent data access without conflicts.
Structuring Your Database
The importance of a well-structured database cannot be overstated. It fundamentally affects how data is stored, queried, and managed. Firebase introduces a NoSQL database paradigm, which means that it's essential to design your data structure in a way that optimizes for its unique strengths.
- Normalized vs. Denormalized Structures: In traditional relational databases, normalization is often encouraged to reduce redundancy. However, with NoSQL databases like Firebase, denormalization can sometimes offer better performance. Evaluate your requirements to decide if you need a normalized structure for your queries or a denormalized one for ongoing performance.
- Nested Structures: Firebase allows for nested data. While it may seem convenient to nest data deeply, this can impair performance for queries. Keeping data somewhat flat can help prevent excessive read times and make data more accessible.
- Employing Collections: Use collections and documents properly to group related data. This structure enables easier data retrieval and enhances organization. For example, if you're building a user management system, separate collections for users, posts, and comments can facilitate clearer relationships.
A well-planned database structure not only aids in maintaining the integrity of data but also decreases the likelihood of encountering issues when scaling.
Handling Concurrent Data
In multi-user environments, especially in real-time applications, handling concurrent data access becomes crucial. Firebase is designed to handle simultaneous connections, but developers must implement thoughtful strategies for managing conflicts that may arise.
- Optimistic Concurrency Control: One common method is to employ optimistic concurrency control, which assumes conflicts are rare. By using timestamps or version numbers, applications can check if the data has changed before committing an update. If a conflict is detected, the application can alert users or merge changes intelligently.
- Transactions: Firebase provides transaction support that allows developers to read and write data atomically, which is essential for ensuring data integrity. Transactions are particularly useful when updating counters or any other data that could be simultaneously modified by different users.
- Data Validation and Security Rules: It is also wise to define data validation rules within Firebase to ensure that concurrent data modifications meet your application requirements. Setting proper security rules can prevent unwanted changes and ensure that only authorized updates are made.
Designing a database and managing concurrent data effectively are vital steps for ensuring operational efficiency and user satisfaction. By committing to these best practices in data management, developers can build robust Firebase and Node.js applications that stand up to the demands of modern usage.
Securing Your Application
In modern application development, security is a crucial aspect that developers cannot overlook. When integrating Firebase with Node.js, securing your application ensures that sensitive data remains protected from unauthorized access, and it helps maintain user trust. This section will discuss important concepts, emphasizing the implementation of security across various levels of the application. The benefits of robust security measures cannot be overstated, as they safeguard both the application and its user data, ultimately leading to a trustworthy digital environment.
Implementing Security Rules
Firebase provides a flexible and powerful system for establishing security rules that control access to your data. These rules are essential for defining who can read and write data within your Firebase services. By implementing security rules, you create a framework that enforces data protection and mitigates risks associated with unauthorized access.
There are several key considerations when setting up security rules:
- Granularity: Apply rules at the smallest unit possible. For instance, rather than setting an overarching rule for a database, target specific entries or collections.
- Role-based Access Control: Differentiate access based on user roles. For instance, administrators may have different access compared to regular users.
- Validation: Validate inputs to ensure that only appropriate data types are accepted.
Here is an example of a simple security rule that only allows authenticated users to write to the database:
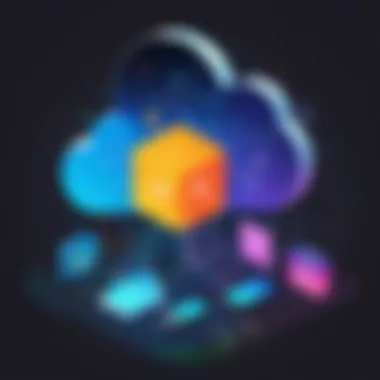
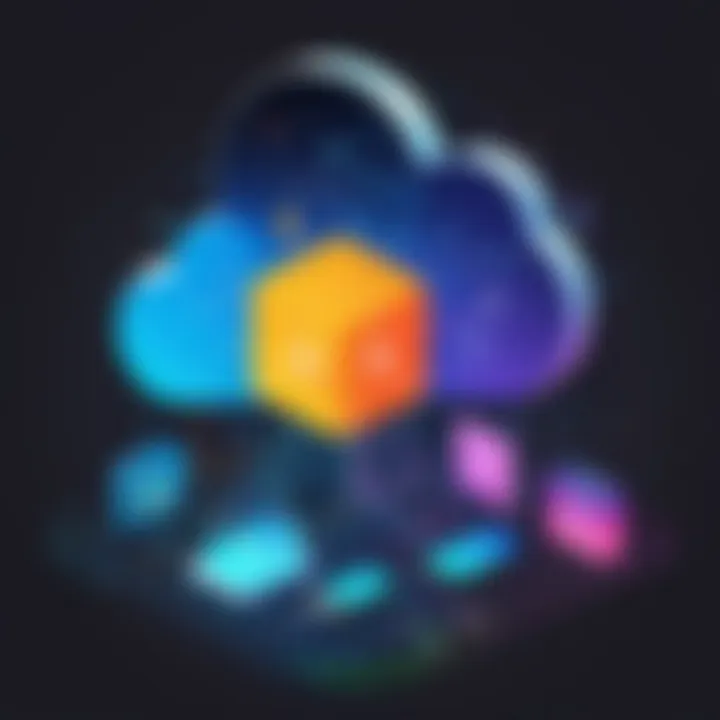
This configuration prevents unauthorized users from modifying data, drastically enhancing security. Therefore, careful planning and implementation of these rules directly impact the safety of your application.
Best Practices for Authentication
Authentication is a fundamental component of application security. When integrating Firebase with Node.js, there are several best practices for implementing authentication effectively. Secure authentication helps ensure that only valid users gain access to your application, thus preserving data integrity and user privacy.
Here are a few best practices to consider:
- Use Strong Password Policies: Encourage users to create complex passwords. This should include a mix of uppercase, lowercase, numeric, and special characters.
- Enable Two-Factor Authentication: Adding a second layer of security can significantly reduce unauthorized access risks.
- Session Management: Properly manage user sessions. This includes tracking login status and providing users the ability to log out, which ensures session termination when needed.
- Regular Audits: Periodically review authentication logs for suspicious activity. Monitoring helps in identifying and rectifying vulnerabilities.
By following these practices, you can enhance application security, making it difficult for attackers to breach your system. Design matters too; the user interface should make it simple and secure for users to log in and manage their accounts without confusion.
"Security is a continuous concern; it must evolve with emerging threats and practices."
In summary, secure applications are a necessity in today's digital landscape. Integrating proper security measurements and best practices into your Firebase and Node.js applications will provide not only a safer environment for users but will also enhance the credibility of your work as a developer.
Performance Optimization
Performance optimization is a critical aspect of any application development process, particularly when working with Firebase and Node.js. The goal is to ensure that applications run efficiently, respond quickly to user commands, and manage resources effectively. Optimizing performance can lead to improved user experience, greater user satisfaction, and reduced operational costs.
When integrating Firebase with Node.js, there are several elements to consider in performance optimization. First, understanding the typical load on your application can aid in formulating strategies to handle high traffic effectively. Recognizing the peak usage times allows developers to optimize database interactions and reduce latency by managing connections appropriately.
Monitoring Performance Metrics
To improve performance, start with monitoring essential performance metrics. This involves collecting and analyzing data that can reveal bottlenecks or areas where improvements can be made.
- Latency: Measure how long it takes for requests to go from the client to the server and back. High latency may indicate network issues or inefficiencies in the codebase.
- Throughput: Assess the number of requests your application can handle in a given time frame. Higher throughput indicates better performance but may require careful management of resources.
- Error Rates: Track the frequency of errors occurring during requests. High error rates can directly impact user satisfaction.
Firebase provides tools like the Firebase Performance Monitoring SDK. This SDK helps track response times and network requests, allowing you to see which operations are slowest and need optimization. Consider integrating tools such as Google Analytics for additional insights into user behavior and application performance.
Optimizing Database Reads/Writes
Database operations often create performance bottlenecks, particularly when reading and writing data. Optimizing these operations can significantly enhance application performance.
- Use Indexed Queries: Instead of querying entire datasets, leverage Firebase's indexing features. By indexing your data, you improve access times and make your application more responsive.
- Limit Data Transferred: Only request the data you need. Using instead of in Firebase Realtime Database can help minimize the amount of data pulled from the server if you donโt need continuous updates.
- Batch Operations: Utilize batch writes for updating multiple documents in one go. This method limits the number of round trips to the server, thus improving write performance.
- Optimize Subscriptions: In real-time applications, managing subscriptions to the database is crucial. Limit the number of listeners attached to database references, and unsubscribe when they are no longer needed.
"Optimization is not about making something perfect; it is about making something good enough to serve its purpose efficiently."
By focusing on these strategies for monitoring and optimizing database reads and writes, developers can enhance the performance of their Firebase and Node.js applications considerably. Ultimately, a well-optimized application not only improves the user experience but also aids in maintaining a sustainable, cost-effective infrastructure.
Common Issues and Troubleshooting
Common issues can arise when integrating Firebase with Node.js. Addressing these issues is vital for ensuring a smooth development process and an optimal user experience. Developers, whether novice or experienced, must anticipate pitfalls and be equipped with strategies to resolve them. This section aims to provide insights into the common problems developers face, focusing on authentication issues and database read/write errors. Understanding these challenges is important to maintain application security and performance.
Resolving Authentication Problems
Authentication issues can stem from various factors, ranging from configuration errors to outdated SDK versions. These problems may prevent users from signing in or accessing specific functionalities of your application. To resolve authentication challenges, follow these steps:
- Check API key and configuration: Ensure that the Firebase configuration settings are correctly applied. Verify that the API key, domain, and project ID are properly set in your Node.js environment. Incorrect configurations will lead to authentication failures.
- Verify user credentials: If authentication fails for specific users, confirm that their credentials are correct. This may involve reviewing the signup and login processes, ensuring that data validation and error handling are properly implemented.
- Examine Firebase Console: Check the Firebase Console for any issues or restrictions on the authentication methods being used. Misconfiguration or constraints on enabled sign-in methods can block authentication attempts.
- Update SDK: Ensure you are using the latest version of the Firebase SDK. Updates often include fixes for known authentication issues. An outdated SDK can lead to unexpected authentication problems.
- Check security rules: Review your Firebase security rules to confirm that they allow the intended actions. Improperly configured rules might restrict access and cause failures in user sign-in processes.
By systematically addressing these authentication concerns, you can enhance the user experience and maintain application effectiveness.
Database Read/Write Errors
Errors related to reading and writing in the Firebase database are not uncommon. These errors can disrupt data flow and hinder application functionality. Common issues include permission errors, data mismatch, and even connectivity problems. To troubleshoot these errors, consider the following steps:
- Review security rules: Security rules govern who can access or modify data. If a user encounters permission denied errors, check if the rules correspond to the intended access patterns. Adjust the rules as necessary.
- Debug data structure: Confirm that the data structure you are using in your Node.js application matches what is defined in Firebase. Inconsistent data formats can lead to read and write failures.
- Check network connectivity: Ensure your application has a stable internet connection. Network issues can result in timeout errors or unresponsive requests, so be sure to test the app under various network conditions.
- Error handling: Implement comprehensive error handling in your code. This allows you to capture and identify errors when they occur, providing you with insight into what might be causing issues.
- Firebase documentation: Consult the official Firebase documentation for insights on specific read/write errors. The documentation provides detailed explanations and solutions for many common problems.
By being proactive in identifying and resolving these common database read and write issues, you can foster a more reliable and seamless application experience. Ensuring the smooth operation of authentication processes and database interactions is essential for the overall success of your application.
Culmination and Future Directions
In the final stages of this guide, it is essential to focus on the significance of the conclusions drawn and the potential future directions for developers working with Firebase and Node.js. The integration of these two platforms can open up numerous possibilities in application development. Being aware of the key takeaways helps in capitalizing on the strengths of both technologies.
Developers who can utilize Firebase within a Node.js environment will find themselves better equipped to create scalable, real-time applications. Emphasizing best practices and optimization techniques will allow these applications to perform efficiently under various loads. Turning attention to security protocols ensures that the applications are not only effective but also safe from unauthorized access and data breaches.
As we look ahead, continuous learning remains a core aspect of development. The technological landscape evolves rapidly, and keeping abreast of new features and updates from Firebase and Node.js is crucial. Following community forums, documentation, and resources will enable developers to stay updated with the latest best practices.
Integrating Firebase with Node.js empowers developers to build dynamic and responsive applications while optimizing performance and security.
Summarizing Key Takeaways
Several points stand as key takeaways from integrating Firebase with Node.js:
- Real-time Capabilities: One of the notable features of Firebase is its real-time database, which allows for instantaneous data synchronization. This is especially valuable in applications requiring live updates, such as messaging apps or collaborative platforms.
- Authentication Made Easy: Firebase offers an array of authentication methods. From email/password to third-party integrations like Google and Facebook, it simplifies user management and increases security.
- Serverless Functions: Utilizing Firebase Cloud Functions offers a way for developers to execute backend code without the need for managing servers. This enables a focus on application logic instead of infrastructure concerns.
- Performance Optimization: Understanding how to monitor and optimize your Firebase and Node.js setup can enhance user experience. Learning to improve database reads/writes is essential for app responsiveness.
Exploring Further Learning Resources
For developers eager to delve deeper, the following resources can provide supporting information and advanced topics related to Firebase and Node.js:
- Firebase Documentation: The official documentation at firebase.google.com is a valuable reference for understanding the complete range of features and functions available in Firebase.
- Node.js Documentation: Similarly, the Node.js documentation found at nodejs.org offers comprehensive insights into the capabilities provided by the platform.
- Community Forums: Engaging with communities on platforms like reddit.com can yield practical tips and share experiences with other developers.
- Books and Online Courses: Considering books such as "Learning Firebase" or online platforms like Coursera and Udemy can help solidify knowledge around application development using these technologies.
With these tools and insights, developers can continue their journey in building powerful applications with Firebase and Node.js, adapting quickly to industry changes.